桌子模型
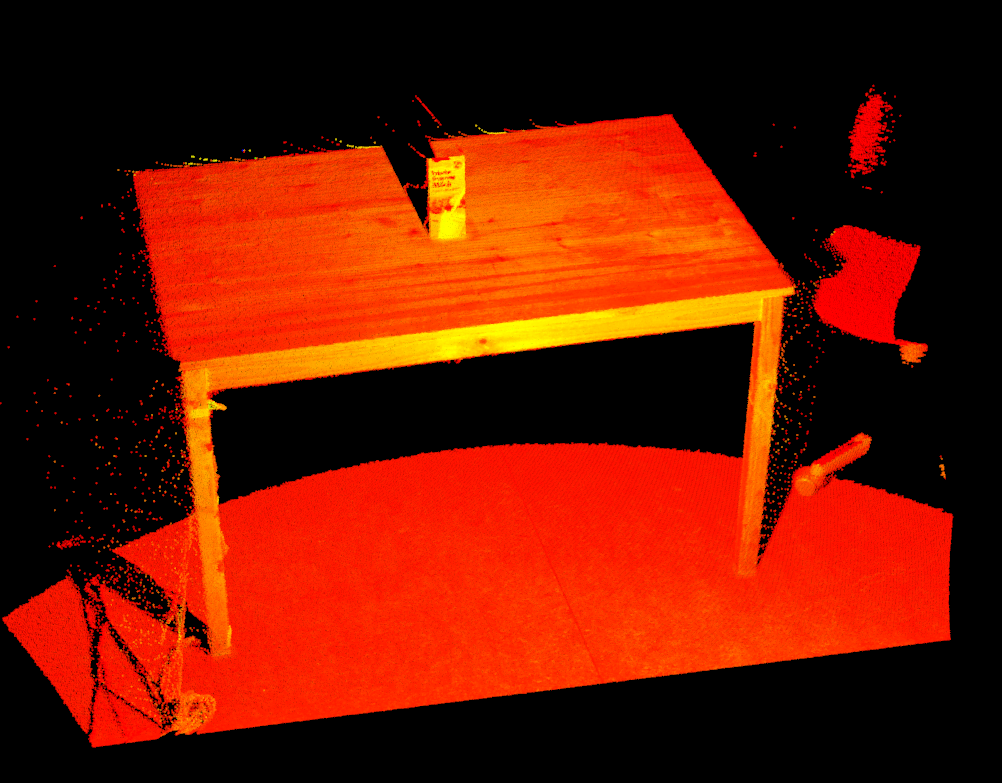
下载:https://raw.github.com/PointCloudLibrary/data/master/tutorials/table_scene_lms400.pcd
代码
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/point_cloud.h>
#include <pcl/visualization/pcl_visualizer.h>
#include <pcl/filters/extract_indices.h>
#include <pcl/segmentation/sac_segmentation.h>
#include <vtkPoints.h>
#include <vtkPolyData.h>
#include <vtkActor.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkInteractorStyle.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkProperty.h>
#include <vtkCellArray.h>
#include <vtkInteractorStyleTrackballCamera.h>
(vtkRenderingOpenGL);
VTK_MODULE_INIT(vtkInteractionStyle);
VTK_MODULE_INIT
// VTK绘制剩下的点云
void drawSegment(pcl::PointIndices &indices, pcl::PointCloud<pcl::PointXYZ>::Ptr cloud)
{
<vtkPoints> points = vtkSmartPointer<vtkPoints>::New();
vtkSmartPointer<vtkCellArray> vertices = vtkSmartPointer<vtkCellArray>::New();
vtkSmartPointerfor (int i: (indices).indices) {
[1];
vtkIdType pid[0] = points->InsertNextPoint(cloud->at(i).x, cloud->at(i).y,
pid->at(i).z);
cloud->InsertNextCell(1, pid);
vertices}
*polyData = vtkPolyData::New();
vtkPolyData ->SetPoints(points);
polyData->SetVerts(vertices);
polyData
*mapper = vtkPolyDataMapper::New();
vtkPolyDataMapper ->SetInputData(polyData);
mapper
*actor = vtkActor::New();
vtkActor ->SetMapper(mapper);
actor->GetProperty()->SetColor(1.0, 0.0, 0.0);
actor->GetProperty()->SetPointSize(3);
actor
*renderer = vtkRenderer::New();
vtkRenderer ->AddActor(actor);
renderer->SetBackground(.0, .0, .0);//set background
renderer
*renderwind = vtkRenderWindow::New();
vtkRenderWindow ->AddRenderer(renderer);
renderwind
*style = vtkInteractorStyleTrackballCamera::New();
vtkInteractorStyleTrackballCamera
*renderwindIt = vtkRenderWindowInteractor::New();
vtkRenderWindowInteractor ->SetRenderWindow(renderwind);
renderwindIt->SetInteractorStyle(style);
renderwindIt
->Render();
renderwind->Start();
renderwindIt}
int main()
{
::PointCloud<pcl::PointXYZ>::Ptr cloud_source(new pcl::PointCloud<pcl::PointXYZ>);
pclstd::string fileName = getenv("HOME") + std::string("/data_set/table_scene_lms400.pcd");
std::cout << fileName << std::endl;
::io::loadPCDFile(fileName, *cloud_source);
pcl// 模型系数
::ModelCoefficients::Ptr coefficients(new pcl::ModelCoefficients);
pcl// 点索引数组
::PointIndices::Ptr inliers(new pcl::PointIndices);
pcl::SACSegmentation<pcl::PointXYZ> sac;
pcl.setInputCloud(cloud_source);
sac// 提取方法
.setMethodType(pcl::SAC_RANSAC);
sac// 提取的物体模型
.setModelType(pcl::SACMODEL_PLANE);
sac.setDistanceThreshold(0.01);
sac.setMaxIterations(100);
sac.setProbability(0.95);
sac.segment(*inliers, *coefficients);
sac// 拟合系数
std::cout << *coefficients << std::endl;
// 绘制分割后的点云
(*inliers, cloud_source);
drawSegment}
运行结果
header:
seq: 0 stamp: 0 frame_id:
values[]
values[0]: -0.00691321
values[1]: -0.875345
values[2]: -0.48345
values[3]: -1.17686
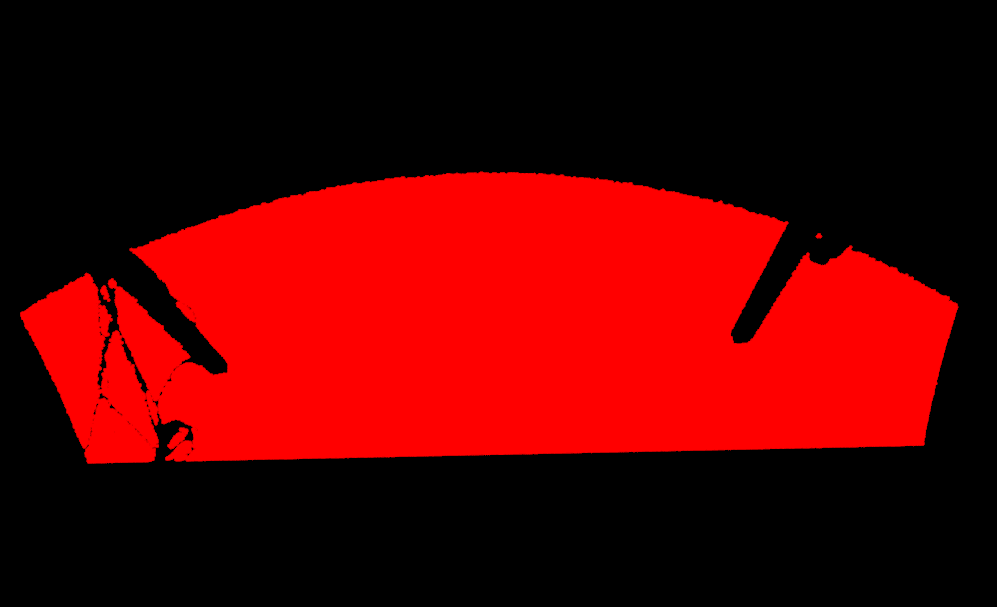
参考
https://pcl.readthedocs.io/projects/tutorials/en/master/planar_segmentation.html#planar-segmentation